public function createPreview(obj:*):BitmapData
{
var resultShirt:BitmapData = new BitmapData(obj.width, obj.height, false, 0xffffff);
var m:Matrix = new Matrix();
var s:Number = resultShirt.height / obj.width;
obj.mask = null;
m.translate(-obj.width / 2, -obj.height / 2);
m.translate(resultShirt.width / 2 , resultShirt.height / 2 );
resultShirt.draw(obj, m, null, null, null, false);
return resultShirt;
}
Export bitmap from MovieClip (DisplayObject)
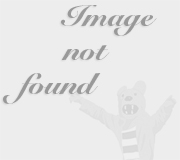
Posted by TodayRatha
on
-
Basic: Papervision3D , Plane and Movieclip Meterail
This is my first Papervision3D. I am starting to learn papervision3d. Download the source if you want to start with Papervision3D:
Uh! sorry Blogger not allow to upload . so please let view the very summary code as below:
package
{
import flash.display.Bitmap;
import flash.display.GradientType;
import flash.display.Graphics;
import flash.display.MovieClip;
import flash.display.Sprite;
import flash.events.Event;
import flash.geom.Matrix;
import org.papervision3d.materials.ColorMaterial;
import org.papervision3d.materials.MovieMaterial;
import org.papervision3d.objects.DisplayObject3D;
import org.papervision3d.objects.primitives.Plane;
import org.papervision3d.view.BasicView;
import AssetManager;
/**
* ...
* @author Ratha
*/
public class Main extends BasicView
{
private var plane:Plane;
public function Main():void
{
super(640, 480);
gradBg();
var mc1:MovieClip = new MovieClip;
var imgClass:Class = AssetManager.MyImg;
var img:Bitmap = new imgClass as Bitmap;
img.smoothing = true;
mc1.addChild(img);
var mat:MovieMaterial = new MovieMaterial(mc1, true, true, true);
mat.smooth = true;
mat.doubleSided = true;
plane = new Plane(mat, 351, 487, 4, 4);
scene.addChild(plane);
camera.zoom = 100;
startRendering();
addEventListener(Event.ENTER_FRAME, makeItMove);
}
private function gradBg():void
{
var g:Graphics = this.graphics;
var gMat:Matrix = new Matrix;
gMat.createGradientBox(this.width, this.height, ( -90 * Math.PI/180)) ;
g.beginGradientFill(GradientType.LINEAR, [0x000000, 0xffffff], [100, 100], [0, 255], gMat);
g.drawRect(0, 0, this.width, this.height);
g.endFill();
}
private function makeItMove(evt:Event):void
{
plane.rotationY += 2;
}
private function init(e:Event = null):void
{
}
}
}
Uh! sorry Blogger not allow to upload . so please let view the very summary code as below:
package
{
import flash.display.Bitmap;
import flash.display.GradientType;
import flash.display.Graphics;
import flash.display.MovieClip;
import flash.display.Sprite;
import flash.events.Event;
import flash.geom.Matrix;
import org.papervision3d.materials.ColorMaterial;
import org.papervision3d.materials.MovieMaterial;
import org.papervision3d.objects.DisplayObject3D;
import org.papervision3d.objects.primitives.Plane;
import org.papervision3d.view.BasicView;
import AssetManager;
/**
* ...
* @author Ratha
*/
public class Main extends BasicView
{
private var plane:Plane;
public function Main():void
{
super(640, 480);
gradBg();
var mc1:MovieClip = new MovieClip;
var imgClass:Class = AssetManager.MyImg;
var img:Bitmap = new imgClass as Bitmap;
img.smoothing = true;
mc1.addChild(img);
var mat:MovieMaterial = new MovieMaterial(mc1, true, true, true);
mat.smooth = true;
mat.doubleSided = true;
plane = new Plane(mat, 351, 487, 4, 4);
scene.addChild(plane);
camera.zoom = 100;
startRendering();
addEventListener(Event.ENTER_FRAME, makeItMove);
}
private function gradBg():void
{
var g:Graphics = this.graphics;
var gMat:Matrix = new Matrix;
gMat.createGradientBox(this.width, this.height, ( -90 * Math.PI/180)) ;
g.beginGradientFill(GradientType.LINEAR, [0x000000, 0xffffff], [100, 100], [0, 255], gMat);
g.drawRect(0, 0, this.width, this.height);
g.endFill();
}
private function makeItMove(evt:Event):void
{
plane.rotationY += 2;
}
private function init(e:Event = null):void
{
}
}
}
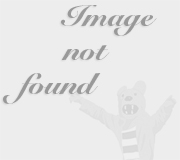
Posted by TodayRatha
on
-
AS3: Short statements
Call function
this[shapes[currentShape]]();
Meaning:
shapes is array of function.
exp: shapes[currentShape] = "drawingLine"
so it mean drawingLine();
Object Name
this['ship' + i]
Meaning:
this is current object. there object named ship1, ship2, ship3, ship4
so in for loop, when i = 2 , it means calling to object ship2.
this[shapes[currentShape]]();
Meaning:
shapes is array of function.
exp: shapes[currentShape] = "drawingLine"
so it mean drawingLine();
Object Name
this['ship' + i]
Meaning:
this is current object. there object named ship1, ship2, ship3, ship4
so in for loop, when i = 2 , it means calling to object ship2.
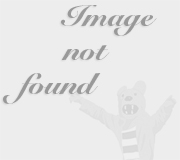
Posted by TodayRatha
on
-
Width and Hieght of Rectangle that fill the Area of inner oval

I don't have the detail formula. I just get the answer by experiment drawing Oval and Rectangle. I measure the width of oval and width of rectangle, then I got the answer as below:
wRec = wCir / 1.41
hRec = hCir / 1.41
It is useful when we want to add the textbox area to an oval without make text show out of the oval.
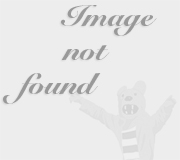
Posted by TodayRatha
on
-
View Simulate Download
I think this is useful if we want to test project with preloader. I may want to see how preloader work.
In Flash Authoring Tool
- Press Ctrl + Enter or Click on Menu Control -> Test Movie.
- Once in Test Movie mode, you can watch a simulated download by enabling the Bandwidth Profiler , by Click on Test Movie Window: View -> Bandwidth Profiler
- Then click on View -> Simulate Download
- You can change the download setting by : View -> Download Setting , then choose the speed of internet.
In FireFox
Check out http://www.dallaway.com/sloppy/
In Flash Authoring Tool
- Press Ctrl + Enter or Click on Menu Control -> Test Movie.
- Once in Test Movie mode, you can watch a simulated download by enabling the Bandwidth Profiler , by Click on Test Movie Window: View -> Bandwidth Profiler
- Then click on View -> Simulate Download
- You can change the download setting by : View -> Download Setting , then choose the speed of internet.
In FireFox
Check out http://www.dallaway.com/sloppy/
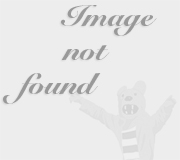
Posted by TodayRatha
on
-
AS3 Multiple Field Array - Sort On
var records:Array = new Array();
records.push({name:"john", city:"omaha", zip:68144});
records.push({name:"john", city:"kansas city", zip:72345});
records.push({name:"bob", city:"omaha", zip:94010});
for(var i:uint = 0; i < records.length; i++) {
trace(records[i].name + ", " + records[i].city);
}
// Results:
// john, omaha
// john, kansas city
// bob, omaha
trace("records.sortOn('name', 'city');");
records.sortOn(["name", "city"]);
for(var i:uint = 0; i < records.length; i++) {
trace(records[i].name + ", " + records[i].city);
}
// Results:
// bob, omaha
// john, kansas city
// john, omaha
trace("records.sortOn('city', 'name');");
records.sortOn(["city", "name"]);
for(var i:uint = 0; i < records.length; i++) {
trace(records[i].name + ", " + records[i].city);
}
// Results:
// john, kansas city
// bob, omaha
records.push({name:"john", city:"omaha", zip:68144});
records.push({name:"john", city:"kansas city", zip:72345});
records.push({name:"bob", city:"omaha", zip:94010});
for(var i:uint = 0; i < records.length; i++) {
trace(records[i].name + ", " + records[i].city);
}
// Results:
// john, omaha
// john, kansas city
// bob, omaha
trace("records.sortOn('name', 'city');");
records.sortOn(["name", "city"]);
for(var i:uint = 0; i < records.length; i++) {
trace(records[i].name + ", " + records[i].city);
}
// Results:
// bob, omaha
// john, kansas city
// john, omaha
trace("records.sortOn('city', 'name');");
records.sortOn(["city", "name"]);
for(var i:uint = 0; i < records.length; i++) {
trace(records[i].name + ", " + records[i].city);
}
// Results:
// john, kansas city
// bob, omaha
// john, omaha
Source: http://livedocs.adobe.com/flash/9.0/ActionScriptLangRefV3/Array.html#sortOn()
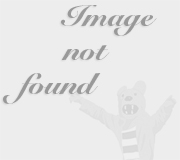
Posted by TodayRatha
on
-
AS3 DataGrid getItemAt Example
var rowCount:Number = dg.length;
trace("[AddUserForm] RowCount is " + rowCount);
selectedItemStr = ""
for (var i:Number = 0 ; i < rowCount ; i++)
{
var obj:Object = dg.getItemAt(i);
if (obj.checked == "true" || obj.checked == true)
{
selectedItemStr += ""; //for now working only project , future
}
}
Code explaination
=============
getItemAt(i)
i is the row index of dataGrid. It start from 0
Return of getItemAt(i) is the Object.
obj = dg.getItemAt(i);
dg is datagrid;
obj is Object
It will return the row from XML of dataProvider. for example
at row i
so if we trace obj.projectID; //out put is 1
trace(obj.projectName); //output is Lorem
trace("[AddUserForm] RowCount is " + rowCount);
selectedItemStr = "
for (var i:Number = 0 ; i < rowCount ; i++)
{
var obj:Object = dg.getItemAt(i);
if (obj.checked == "true" || obj.checked == true)
{
selectedItemStr += "
}
}
Code explaination
=============
getItemAt(i)
i is the row index of dataGrid. It start from 0
Return of getItemAt(i) is the Object.
obj = dg.getItemAt(i);
dg is datagrid;
obj is Object
It will return the row from XML of dataProvider. for example
so if we trace obj.projectID; //out put is 1
trace(obj.projectName); //output is Lorem
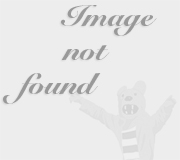
Posted by TodayRatha
on
-
AS3: Load fresh XML without Caching.
I found the problem, and I try to found on the internet. Some poster commanded using this:
var Randomstr:String = '?somvarname='+random(100);
I don't know, but it does not for me. so I use this instead of:
var iRequestXML:URLRequest = new URLRequest("images/" + editData);
var iLoaderXML:URLLoader = new URLLoader;
var iVaXML:URLVariables = new URLVariables;
iVaXML.noCach = int(Math.random() * 999);
iRequestXML.method = URLRequestMethod.POST;
iRequestXML.data = iVaXML;
iLoaderXML.addEventListener(Event.COMPLETE, loadXMLEdit);
iLoaderXML.addEventListener(IOErrorEvent.IO_ERROR, loadXMLError);
iLoaderXML.load(iRequestXML);
Use URLVariable instead of use query string.
var Randomstr:String = '?somvarname='+random(100);
I don't know, but it does not for me. so I use this instead of:
var iRequestXML:URLRequest = new URLRequest("images/" + editData);
var iLoaderXML:URLLoader = new URLLoader;
var iVaXML:URLVariables = new URLVariables;
iVaXML.noCach = int(Math.random() * 999);
iRequestXML.method = URLRequestMethod.POST;
iRequestXML.data = iVaXML;
iLoaderXML.addEventListener(Event.COMPLETE, loadXMLEdit);
iLoaderXML.addEventListener(IOErrorEvent.IO_ERROR, loadXMLError);
iLoaderXML.load(iRequestXML);
Use URLVariable instead of use query string.
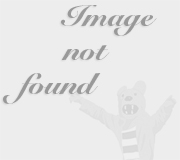
Posted by TodayRatha
on
-
AS3: Clone or Duplicate Movie
private function cloneDpObj(target:DisplayObject):DisplayObject
{
// create duplicate
var targetClass:Class = Object(target).constructor;
trace("targetClass::" + targetClass);
var duplicate:DisplayObject = new targetClass();
// duplicate properties
duplicate.transform = target.transform;
duplicate.filters = target.filters;
duplicate.cacheAsBitmap = target.cacheAsBitmap;
duplicate.opaqueBackground = target.opaqueBackground;
if (target.scale9Grid) {
var rect:Rectangle = target.scale9Grid;
// WAS Flash 9 bug where returned scale9Grid is 20x larger than assigned
// rect.x /= 20, rect.y /= 20, rect.width /= 20, rect.height /= 20;
duplicate.scale9Grid = rect;
}
// add to target parent's display list
// if autoAdd was provided as true
return duplicate;
}
{
// create duplicate
var targetClass:Class = Object(target).constructor;
trace("targetClass::" + targetClass);
var duplicate:DisplayObject = new targetClass();
// duplicate properties
duplicate.transform = target.transform;
duplicate.filters = target.filters;
duplicate.cacheAsBitmap = target.cacheAsBitmap;
duplicate.opaqueBackground = target.opaqueBackground;
if (target.scale9Grid) {
var rect:Rectangle = target.scale9Grid;
// WAS Flash 9 bug where returned scale9Grid is 20x larger than assigned
// rect.x /= 20, rect.y /= 20, rect.width /= 20, rect.height /= 20;
duplicate.scale9Grid = rect;
}
// add to target parent's display list
// if autoAdd was provided as true
return duplicate;
}
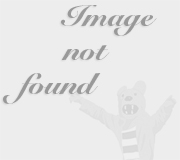
Posted by TodayRatha
on
-
AS3 : Basic FLV Player
More resource may check here:
http://www.adobe.com/livedocs/flash/9.0/ActionScriptLangRefV3/flash/media/Video.html
http://www.adobe.com/livedocs/flash/9.0/ActionScriptLangRefV3/flash/net/NetConnection.html
AS3 Simple:
package {
import flash.display.Sprite;
import flash.display.MovieClip;
import flash.net.NetConnection;
import flash.net.NetStream;
import flash.media.Video;
public class main extends MovieClip {
private var nc:NetConnection;
private var ns:NetStream;
private var vid:Video;
private var client:Object;
public function main () {
// Initialize net stream
nc = new NetConnection();
nc.connect (null); // Not using a media server.
ns = new NetStream(nc);
// Add video to stage
vid = new Video(320,240);
addChild (vid);
//vid.x = ( stage.stageWidth / 2) - ( vid.width / 2 );
//vid.y = ( stage.stageHeight / 2) - ( vid.height / 2 );
// Changed since when deployed the
// above set the video player nearly off the screen
// Since I am lazy, I am just going to 0 them
// out for now. Apparently, I have a lot more
// to learn.
vid.x = 0;
vid.y = 0;
// Add callback method for listening on
// NetStream meta data
client = new Object();
ns.client = client;
client.onMetaData = nsMetaDataCallback;
// Play video
vid.attachNetStream ( ns );
ns.play ( 'test FLV.flv' );
}
//MetaData
private function nsMetaDataCallback (mdata:Object):void {
trace (mdata.duration);
}
}
}
Check if video is finish
================
http://www.adobe.com/livedocs/flash/9.0/ActionScriptLangRefV3/flash/media/Video.html
http://www.adobe.com/livedocs/flash/9.0/ActionScriptLangRefV3/flash/net/NetConnection.html
AS3 Simple:
package {
import flash.display.Sprite;
import flash.display.MovieClip;
import flash.net.NetConnection;
import flash.net.NetStream;
import flash.media.Video;
public class main extends MovieClip {
private var nc:NetConnection;
private var ns:NetStream;
private var vid:Video;
private var client:Object;
public function main () {
// Initialize net stream
nc = new NetConnection();
nc.connect (null); // Not using a media server.
ns = new NetStream(nc);
// Add video to stage
vid = new Video(320,240);
addChild (vid);
//vid.x = ( stage.stageWidth / 2) - ( vid.width / 2 );
//vid.y = ( stage.stageHeight / 2) - ( vid.height / 2 );
// Changed since when deployed the
// above set the video player nearly off the screen
// Since I am lazy, I am just going to 0 them
// out for now. Apparently, I have a lot more
// to learn.
vid.x = 0;
vid.y = 0;
// Add callback method for listening on
// NetStream meta data
client = new Object();
ns.client = client;
client.onMetaData = nsMetaDataCallback;
// Play video
vid.attachNetStream ( ns );
ns.play ( 'test FLV.flv' );
}
//MetaData
private function nsMetaDataCallback (mdata:Object):void {
trace (mdata.duration);
}
}
}
Check if video is finish
================
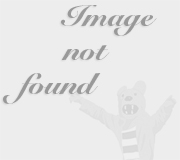
Posted by TodayRatha
on
-
Import Animated Gif to Frame in Photoshop
- Make sure QuickTime 7 or later is installed on your computer.
It is most likely installed during Adobe Photoshop or Adobe CS3 setup but can also be downloaded and installed from Apple web site. - From the File menu, open Import submenu and
select Video Frames to Layers… command.
The Load dialog box shows up. (This will take more than two seconds the first time but appears immediately afterwards.) - Navigate to the folder which contains your Animated GIF files.
They will not show up on your Load dialog box. - In the File Name field, type *.gif and press ENTER.
Your animated GIF files will show up. Select the file you intend to import and click Load button or press ENTER.
Import Video To Layers dialog box will show up.- Ensure the Make Frame Animation check box is checked.
- Click OK button.
The animation will load into Adobe Photoshop.
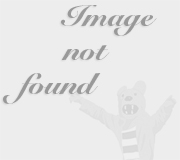
Posted by TodayRatha
on
-
as3 : Get full homepage url
eg: http://localhost:8080
function getHomeURL():String
{
var homeURL:String;
var port:String = ExternalInterface.call('window.location.port.toString');
var hostname:String = ExternalInterface.call('window.location.hostname.toString');
if(port && port!="")
{
port = ":" + port;
}
try
{
homeURL = ExternalInterface.call('window.location.protocol.toString') + "//" + hostname + port;
}
catch(Er:Error)
{
homeURL = "error";
}
return homeURL;
}
function getHomeURL():String
{
var homeURL:String;
var port:String = ExternalInterface.call('window.location.port.toString');
var hostname:String = ExternalInterface.call('window.location.hostname.toString');
if(port && port!="")
{
port = ":" + port;
}
try
{
homeURL = ExternalInterface.call('window.location.protocol.toString') + "//" + hostname + port;
}
catch(Er:Error)
{
homeURL = "error";
}
return homeURL;
}
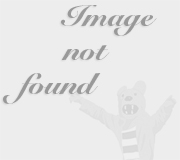
Posted by TodayRatha
on
-
My First AIR App : Facebook Avatar Maker
Facebook Avatar Maker.

Click on browse you photo, then your photo appear on app under frame, drag or zoom it to match your need, then click save, don't forget put the (.jpg) after your file name.
Next version:
- Add more frame style like: Drop water, Heart sign, and more
Download and Install:
- you will need Adobe Air Player to install this app, please get it from http://get.adobe.com/air/ [15.12MB]
- Download Facebook Avatar Maker.air from http://www.mediafire.com/?nj0yklm0jqe
Lol :)

Click on browse you photo, then your photo appear on app under frame, drag or zoom it to match your need, then click save, don't forget put the (.jpg) after your file name.
Next version:
- Add more frame style like: Drop water, Heart sign, and more
Download and Install:
- you will need Adobe Air Player to install this app, please get it from http://get.adobe.com/air/ [15.12MB]
- Download Facebook Avatar Maker.air from http://www.mediafire.com/?nj0yklm0jqe
Lol :)
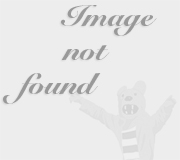
Posted by TodayRatha
on
-
jailbreak iphone/ipod touch 3G MC Model
This is just my note:
1- Download BlackRa1n from Blackweather.com or google it
2- Download iTune any version and install it on PC.
3- Double-click on BlackRa1n , app start, click on make it rain, then wait few second, on screen of ur iphone show BlackRa1n.
4- then if you lucky you can see BlackRa1n icon on your iphone, Make sure you connect to internet with WiFi
5- touch on BlackRa1n icon to run it. if you have internet you will see unless Cydia appear on the list, don't wait, touch on Cydia to install, it would take a while depend on your internet connection
6- after you install Cydia your iphone may reboot, then it will show image to connect your iphone to itune, and on your PC, itune may alert you to restore it. Don't panic, run BlackRa1n to force your iphone run into lock screen (click make it rain, wait for few minute, you will go to logon screen).
7- on Iphone, go to Cydia and intall BossPref ( or SBSettings ) and OpenSSH, if your iphone reboot, don't forget BlackRa1n. (you can go to search and type )
8 -on Iphone, add source to cydia by run cydia, goto Manage, goto Source, touch edit, touch add, type this address: http://cydia.hackulo.us . click ok, anyway till in done.
9- on Iphone, goto search, type : AppSync for OS and then you will see two result: the end is show the version for your firmware, if your firmware is 3.1.3 or 3.1.2, please select it depend on your firmware (to check your firmware, on Iphone, goto setting>general>about look down see firmware).
10, ok- install it,
11- Restart your iphone
12- Welcome your iphone start with BlackRa1n.
13- Google to find Cracked IPA , then double-click on it, you will go to Itune, then you can syn it to your iphone.
14- let enjoy it, if you are rich do not do the jailbreak, please support Apple Policy, buy App from Itune store to support developer, keep them create new app, and game for you.
I like and support techonolgy of Apple, I do the jailbreak to my ipod, because I don't have fast internet to download app from itune store, and I don't use master card.
However, please don't do this, if you have money to buy app from itune store.
1- Download BlackRa1n from Blackweather.com or google it
2- Download iTune any version and install it on PC.
3- Double-click on BlackRa1n , app start, click on make it rain, then wait few second, on screen of ur iphone show BlackRa1n.
4- then if you lucky you can see BlackRa1n icon on your iphone, Make sure you connect to internet with WiFi
5- touch on BlackRa1n icon to run it. if you have internet you will see unless Cydia appear on the list, don't wait, touch on Cydia to install, it would take a while depend on your internet connection
6- after you install Cydia your iphone may reboot, then it will show image to connect your iphone to itune, and on your PC, itune may alert you to restore it. Don't panic, run BlackRa1n to force your iphone run into lock screen (click make it rain, wait for few minute, you will go to logon screen).
7- on Iphone, go to Cydia and intall BossPref ( or SBSettings ) and OpenSSH, if your iphone reboot, don't forget BlackRa1n. (you can go to search and type )
8 -on Iphone, add source to cydia by run cydia, goto Manage, goto Source, touch edit, touch add, type this address: http://cydia.hackulo.us . click ok, anyway till in done.
9- on Iphone, goto search, type : AppSync for OS and then you will see two result: the end is show the version for your firmware, if your firmware is 3.1.3 or 3.1.2, please select it depend on your firmware (to check your firmware, on Iphone, goto setting>general>about look down see firmware).
10, ok- install it,
11- Restart your iphone
12- Welcome your iphone start with BlackRa1n.
13- Google to find Cracked IPA , then double-click on it, you will go to Itune, then you can syn it to your iphone.
14- let enjoy it, if you are rich do not do the jailbreak, please support Apple Policy, buy App from Itune store to support developer, keep them create new app, and game for you.
I like and support techonolgy of Apple, I do the jailbreak to my ipod, because I don't have fast internet to download app from itune store, and I don't use master card.
However, please don't do this, if you have money to buy app from itune store.
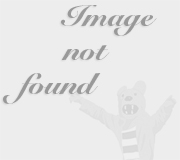
Posted by TodayRatha
on
-
PHP Send Copy Mail
Is it possible (I'm sure it must be) for a copy of the same email to be sent to the person who has just filled in the form?
|
Not a big deal, but if you want to hide the fact the email was copied to someone else, you can do the following:
$headers .= "Bcc: $email\r\n";
copy from Habtom 's post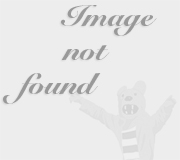
Posted by TodayRatha
on
-
Mootool | Native Array Sum Value in Elements
calculatedTotalCost: function (subTotals)
{
var x = {subValue: 0};
subTotals.each(function(el, i) {
if(el.value)
{
this.subValue+= el.value;
}
}.bind(x));
var updateTotalCost = x.subValue;
this.totalCost.set('text', this.formatCurrency(updateTotalCost.toFixed(2)));
this.totalCost.set('value', updateTotalCost);
},
{
var x = {subValue: 0};
subTotals.each(function(el, i) {
if(el.value)
{
this.subValue+= el.value;
}
}.bind(x));
var updateTotalCost = x.subValue;
this.totalCost.set('text', this.formatCurrency(updateTotalCost.toFixed(2)));
this.totalCost.set('value', updateTotalCost);
},
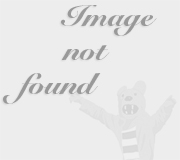
Posted by TodayRatha
on
-
AS3 Convert MovieClip or Display to BitmapData
var shirtImage:BitmapData = new BitmapData(530, 300);
shirtImage.draw(shirtObj);
var imagePreview:MovieClip = new MovieClip();
imagePreview.graphics.beginBitmapFill(shirtImage);
imagePreview.graphics.drawRect(0, 0, 530, 300);
imagePreview.graphics.endFill();
var disableMC:MovieClip = new MovieClip();
layer2.addChild(disableMC);
disableMC.graphics.beginFill(0x000000, 0.75);
disableMC.graphics.drawRect(0, 0, 859, 352);
disableMC.graphics.endFill();
layer1.enabled = false;
TweenMax.to(layer1, 0.25, { blurFilter: { blurX:7, blurY:7, quality:1 }} );
layer2.addChild(imagePreview);
imagePreview.x = 150;
imagePreview.y = 20;
imagePreview.addEventListener(MouseEvent.CLICK, function ()
{
TweenMax.to(layer1, 0.25, { blurFilter: { blurX:0, blurY:0, quality:1 }} );
while( layer2.numChildren > 0 )
layer2.removeChildAt( 0 );
}
);
shirtImage.draw(shirtObj);
var imagePreview:MovieClip = new MovieClip();
imagePreview.graphics.beginBitmapFill(shirtImage);
imagePreview.graphics.drawRect(0, 0, 530, 300);
imagePreview.graphics.endFill();
var disableMC:MovieClip = new MovieClip();
layer2.addChild(disableMC);
disableMC.graphics.beginFill(0x000000, 0.75);
disableMC.graphics.drawRect(0, 0, 859, 352);
disableMC.graphics.endFill();
layer1.enabled = false;
TweenMax.to(layer1, 0.25, { blurFilter: { blurX:7, blurY:7, quality:1 }} );
layer2.addChild(imagePreview);
imagePreview.x = 150;
imagePreview.y = 20;
imagePreview.addEventListener(MouseEvent.CLICK, function ()
{
TweenMax.to(layer1, 0.25, { blurFilter: { blurX:0, blurY:0, quality:1 }} );
while( layer2.numChildren > 0 )
layer2.removeChildAt( 0 );
}
);
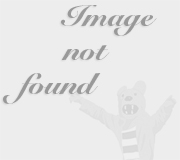
Posted by TodayRatha
on
-
AS3 | Crop image
var cropedImage:BitmapData = new BitmapData(positionImage.width, positionImage.height);
var matrix:Matrix = new Matrix;
var matrixXY:Point = new Point();
var smooth:Boolean = false;
var bounds1 = positionImageMasking.getRect(this);
var bounds2 = positionImage.getRect(this);
matrixXY.x = (bounds1.x - bounds2.x)/positionImageMasking.scaleX;
matrixXY.y = (bounds1.y - bounds2.y)/positionImageMasking.scaleY;
trace("matrixXY.x = " + matrixXY.x);
matrix.tx = matrixXY.x;
matrix.ty = matrixXY.y;
matrix.scale(positionImageMasking.scaleX, positionImageMasking.scaleY);
//matrix.rotate(Math.PI/14);
if (positionImageMasking.scaleX != 1)
{
smooth = true;
}
cropedImage.draw(loadedBitmap.bitmapData, matrix,null,null,null,smooth);
var mc:MovieClip = new MovieClip();
mc.graphics.beginBitmapFill(cropedImage);
mc.graphics.drawRect(0, 0, positionImage.width, positionImage.height);
mc.graphics.endFill();
addChild(mc);
var matrix:Matrix = new Matrix;
var matrixXY:Point = new Point();
var smooth:Boolean = false;
var bounds1 = positionImageMasking.getRect(this);
var bounds2 = positionImage.getRect(this);
matrixXY.x = (bounds1.x - bounds2.x)/positionImageMasking.scaleX;
matrixXY.y = (bounds1.y - bounds2.y)/positionImageMasking.scaleY;
trace("matrixXY.x = " + matrixXY.x);
matrix.tx = matrixXY.x;
matrix.ty = matrixXY.y;
matrix.scale(positionImageMasking.scaleX, positionImageMasking.scaleY);
//matrix.rotate(Math.PI/14);
if (positionImageMasking.scaleX != 1)
{
smooth = true;
}
cropedImage.draw(loadedBitmap.bitmapData, matrix,null,null,null,smooth);
var mc:MovieClip = new MovieClip();
mc.graphics.beginBitmapFill(cropedImage);
mc.graphics.drawRect(0, 0, positionImage.width, positionImage.height);
mc.graphics.endFill();
addChild(mc);
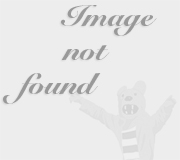
Posted by TodayRatha
on
-
Flash AS3 | Open local Image
import flash.net.FileReference;
public function LocalFileAccessExample():void {
var fileRef = new FileReference();
fileRef.addEventListener( Event.SELECT, onFileSelect );
fileRef.addEventListener( Event.OPEN, onFileOpen );
fileRef.browse();
}
private function onFileSelect( event:Event ):void {
var fileRef:FileReference = event.target as FileReference;
fileRef.open();
}
//--------------------- option with filter
var imagesFilter:FileFilter = new FileFilter("Images",
"*.jpg;*.gif;*.png");
var docFilter:FileFilter = new FileFilter("Documents",
"*.pdf;*.doc;*.txt");
var myFileReference:FileReference = new FileReference();
myFileReference.browse([imagesFilter, docFilter]);
private function onFileOpen( event:Event ):void {
var fileRef:FileReference = event.target as FileReference;
var data:ByteArray = fileRef.data as ByteArray;
}
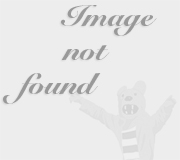
Posted by TodayRatha
on
-
Apache | Virtual Host Configuration
1- Enable Virtual Host in Apache httpd.conf
Start->Programs->Apache HTTP Server->Configure Apache Server->Edit the Apache httpd.conf Configuration File
or goto httpd.conf folder of Apache Installed Folder
Find this
2- Folder Security
Still in httpd.conf
Find where similar of this code are:
3- Create Virtual Host in
Start->Programs->Apache HTTP Server->Configure Apache Server->Edit the Apache httpd.conf Configuration File
or goto httpd.conf folder of Apache Installed Folder
Find this
#Include conf/extra/httpd-vhosts.conf (Ctrl + F, type this, Enter)
Then remove # from the line
so it should be
Include conf/extra/httpd-vhosts.conf
2- Folder Security
Still in httpd.conf
Find where similar of this code are:
then put this element after existed one:
Note: c:\My sites is the path of your website[Directory "C:\ My Sites "]
Order Deny,Allow
Allow from all
[/Directory]
3- Create Virtual Host in
httpd-vhosts.conf
open httpd-vhosts.conf then put this element at the bottom
[VirtualHost 127.0.0.1]
DocumentRoot "C:\My Sites\Site1"
ServerName site1.local
[/VirtualHost]
4- Resolving the DNS issue
goto
C:\WINNT\system32\drivers\etc\hosts
or
C:\Windows\system32\drivers\etc\hosts
Put this code in
127.0.0.1 site1.local
Finally restart apache
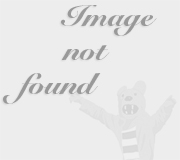
Posted by TodayRatha
on
-
AS3 Retrieve Flash Vars & HTML put Flash (swfObject)
Retrieve Flash Vars
---------------------
------------------------------
Use swfObject can be download here http://code.google.com/p/swfobject/downloads/list
<head>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />
<title>LandingPage2</title>
<script type="text/javascript" src="swfobject.js"></script>
<script type="text/javascript">
var flashvars = {
cicadaUrl:'http://cicadagroup.com.au/index.php?categoryid=1',
fireflyUrl:' ',
chocolateSoldierUrl:' ',
greengrocerUrl:' ',
contactusUrl:' ',
locationUrl:' ',
employmentUrl:' '
};
var params = {
menu: "false"
};
swfobject.embedSWF("LandingPage2.swf", "myContent", "980", "600", "9.0.0","expressInstall.swf", flashvars, params);
</script>
</head>
---------------------
var fileName:String = new String();HTML (swfObject) Flash Vars
fileName = root.loaderInfo.parameters.fileName
------------------------------
Use swfObject can be download here http://code.google.com/p/swfobject/downloads/list
<head>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />
<title>LandingPage2</title>
<script type="text/javascript" src="swfobject.js"></script>
<script type="text/javascript">
var flashvars = {
cicadaUrl:'http://cicadagroup.com.au/index.php?categoryid=1',
fireflyUrl:' ',
chocolateSoldierUrl:' ',
greengrocerUrl:' ',
contactusUrl:' ',
locationUrl:' ',
employmentUrl:' '
};
var params = {
menu: "false"
};
swfobject.embedSWF("LandingPage2.swf", "myContent", "980", "600", "9.0.0","expressInstall.swf", flashvars, params);
</script>
</head>
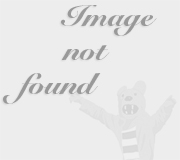
Posted by TodayRatha
on
-
Flash AS3 | Load External Images in Flash vr. 2
import flash.net.URLRequest;
import flash.display.Loader;
import flash.events.Event;
import flash.events.ProgressEvent;
function startLoad()
{
var mLoader:Loader = new Loader();
var mRequest:URLRequest = new URLRequest(“MouseActions.swf”);
mLoader.contentLoaderInfo.addEventListener(Event.COMPLETE, onCompleteHandler);
mLoader.contentLoaderInfo.addEventListener(ProgressEvent.PROGRESS, onProgressHandler);
mLoader.load(mRequest);
}
function onCompleteHandler(loadEvent:Event)
{
addChild(loadEvent.currentTarget.content);
}
function onProgressHandler(mProgress:ProgressEvent)
{
var percent:Number = mProgress.bytesLoaded/mProgress.bytesTotal;
trace(percent);
}
startLoad();
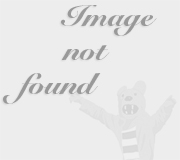
Posted by TodayRatha
on
-
AS3 Flash | Load External XML with urlLoader
AS3
===
var xmlLoader:URLLoader = new URLLoader();
xmlLoader.addEventListener(Event.COMPLETE, showXML);
xmlLoader.load(new URLRequest("playlistAS3.xml"));
funciton
========
function showXML(e:Event):void {
XML.ignoreWhitespace = true;
var songs:XML = new XML(e.target.data);
trace(songs.track.length());
var i:Number;
for (i=0; i < songs.track.length(); i++) {
trace(" Name of the song: "+ songs.track[i].title.text());
trace(" Name of the Band: "+ songs.track[i].band.text());
trace(" File Location of the song: "+ songs.track[i].file.text());
}
}
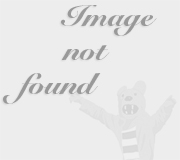
Posted by TodayRatha
on
-
AS3 | Array
Create Array
var myArray:Array = new Array("A", "B", "C");
Using element of an Array
trace(myArray[2]);
output is: C
Adding element to array
1- myArray[3] = "D"; // create element at 3th site of the array
2- myArray.push("D"); //create element after the last site of the array
Changing element of the array
myArray[3] = "Changed D"
(to be continue)
var myArray:Array = new Array("A", "B", "C");
Using element of an Array
trace(myArray[2]);
output is: C
Adding element to array
1- myArray[3] = "D"; // create element at 3th site of the array
2- myArray.push("D"); //create element after the last site of the array
Changing element of the array
myArray[3] = "Changed D"
(to be continue)
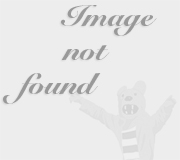
Posted by TodayRatha
on
-
Category List
- Actionscript 3 (2)
- Digital Painting (1)
- Life style (1)
- Photoshop (1)
- Sencha Touch (1)
Blog Archive
-
▼
2010
(50)
-
►
March
(12)
- Mootool | Native Array Sum Value in Elements
- Identify Font
- AS3 | Call Javascript Function
- AS3 | Stage no scale
- Flash AS3 | Why buttonMode not working
- AS3 Convert MovieClip or Display to BitmapData
- AS3 | Crop image
- Flash AS3 | Proportion Width and ScaleX
- Wamp & Another Package PHP | Virtual Host
- PHP | Config with Apache 2.2
- Flash AS3 | Open local Image
- Flash AS3 | Remove all child from display object
-
►
February
(9)
- Flash AS3 | Get Actual Width and Hight of Rotated ...
- Flash AS3 | Get Width and Height of Rotated Object
- Apache | Virtual Host Configuration
- AS3 Retrieve Flash Vars & HTML put Flash (swfObject)
- Flash AS3 | XML return value with the name of node
- Flash AS3 | Draw Squar
- Flash AS3 | Change color of movieclip with TINT
- Flash AS3 | Load External Images in Flash vr. 2
- Flash AS3 | Loading External Images in Flash
-
►
January
(8)
- AS3 Flash | Give result to XML variable
- AS3 Flash | Load External XML with urlLoader
- AS3 Flash | Load External XML with urlLoader
- AS3 Flash | Dispatchevent
- AS3 Flash | Automatically Action using Timer Class
- AS3 Flash | Coding Object have shadow effect
- AS3 | Array
- AS3 Flash | Dynamic Textfield autoSize
-
►
March
(12)